mirror of
https://github.com/red0124/ssp.git
synced 2025-01-22 20:55:18 +01:00
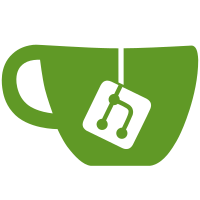
* Bugfix/odr violations (#47) * Make common non-member functions inline, remove unreachable line from get_line_buffer * [skip ci] Fix namespace comments * Resolve clang-tidy warnings (#48) * Resolve clang-tidy warnings, update single_header_generator.py * Update single header test, resolve additional clang-tidy warnings * Add header and raw_header methods, update header usage methods error handling, write new and update existing unit tests * Update parser error messages, fix parser tests * Add [[nodiscard]] where fitting, update unit tests (#49) * Add const where fitting, make splitter class members private, add #pragma once to ssp.hpp * Modify header parsing for empty headers, update old and add new tests for header parsing * Enable the parser to accept a header with one empty field, update unit tests * Fix test CMakeLists.txt typo
128 lines
2.4 KiB
C++
128 lines
2.4 KiB
C++
#pragma once
|
|
|
|
namespace ss {
|
|
|
|
////////////////
|
|
// all except
|
|
////////////////
|
|
|
|
template <typename T, auto... Values>
|
|
struct ax {
|
|
private:
|
|
template <auto X, auto... Xs>
|
|
[[nodiscard]] bool ss_valid_impl(const T& x) const {
|
|
if constexpr (sizeof...(Xs) != 0) {
|
|
return x != X && ss_valid_impl<Xs...>(x);
|
|
}
|
|
return x != X;
|
|
}
|
|
|
|
public:
|
|
[[nodiscard]] bool ss_valid(const T& value) const {
|
|
return ss_valid_impl<Values...>(value);
|
|
}
|
|
|
|
[[nodiscard]] const char* error() const {
|
|
return "value excluded";
|
|
}
|
|
};
|
|
|
|
////////////////
|
|
// none except
|
|
////////////////
|
|
|
|
template <typename T, auto... Values>
|
|
struct nx {
|
|
private:
|
|
template <auto X, auto... Xs>
|
|
[[nodiscard]] bool ss_valid_impl(const T& x) const {
|
|
if constexpr (sizeof...(Xs) != 0) {
|
|
return x == X || ss_valid_impl<Xs...>(x);
|
|
}
|
|
return x == X;
|
|
}
|
|
|
|
public:
|
|
[[nodiscard]] bool ss_valid(const T& value) const {
|
|
return ss_valid_impl<Values...>(value);
|
|
}
|
|
|
|
[[nodiscard]] const char* error() const {
|
|
return "value excluded";
|
|
}
|
|
};
|
|
|
|
////////////////
|
|
// greater than or equal to
|
|
// greater than
|
|
// less than
|
|
// less than or equal to
|
|
////////////////
|
|
|
|
template <typename T, auto N>
|
|
struct gt {
|
|
[[nodiscard]] bool ss_valid(const T& value) const {
|
|
return value > N;
|
|
}
|
|
};
|
|
|
|
template <typename T, auto N>
|
|
struct gte {
|
|
[[nodiscard]] bool ss_valid(const T& value) const {
|
|
return value >= N;
|
|
}
|
|
};
|
|
|
|
template <typename T, auto N>
|
|
struct lt {
|
|
[[nodiscard]] bool ss_valid(const T& value) const {
|
|
return value < N;
|
|
}
|
|
};
|
|
|
|
template <typename T, auto N>
|
|
struct lte {
|
|
[[nodiscard]] bool ss_valid(const T& value) const {
|
|
return value <= N;
|
|
}
|
|
};
|
|
|
|
////////////////
|
|
// in range
|
|
////////////////
|
|
|
|
template <typename T, auto Min, auto Max>
|
|
struct ir {
|
|
[[nodiscard]] bool ss_valid(const T& value) const {
|
|
return value >= Min && value <= Max;
|
|
}
|
|
};
|
|
|
|
////////////////
|
|
// out of range
|
|
////////////////
|
|
|
|
template <typename T, auto Min, auto Max>
|
|
struct oor {
|
|
[[nodiscard]] bool ss_valid(const T& value) const {
|
|
return value < Min || value > Max;
|
|
}
|
|
};
|
|
|
|
////////////////
|
|
// non empty
|
|
////////////////
|
|
|
|
template <typename T>
|
|
struct ne {
|
|
[[nodiscard]] bool ss_valid(const T& value) const {
|
|
return !value.empty();
|
|
}
|
|
|
|
[[nodiscard]] const char* error() const {
|
|
return "empty field";
|
|
}
|
|
};
|
|
|
|
} /* namespace ss */
|